Understanding Selenium WebDriver: Key Concepts and Features
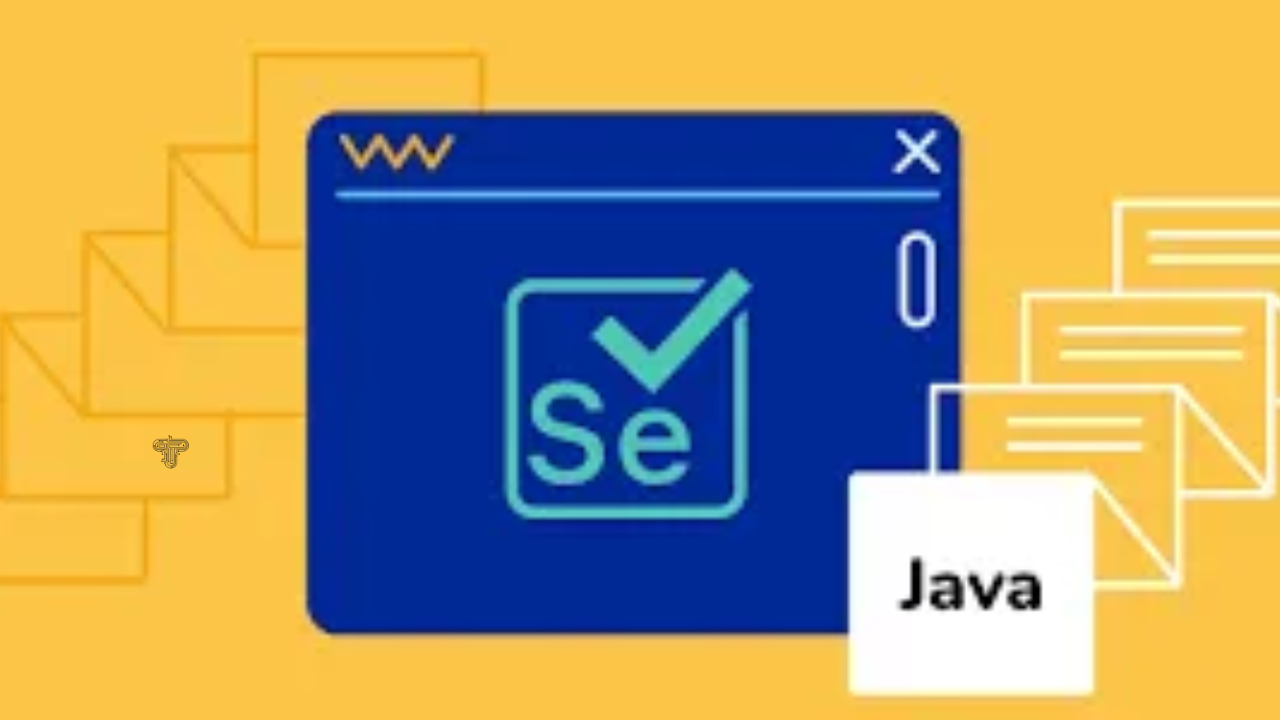
Selenium WebDriver is one of the most famous web application UI testing instruments. It offers a good tool for automating browsers to reduce testing time and easily perform cross-testing from the developer and tester side. In this guide, we will try to give an idea about the fundamental idea behind what is Selenium WebDriver, insight, and approaches to use it effectively and efficiently.
What is Selenium WebDriver?
Selenium WebDriver is an automation tool for interacting with browsers, part of the Selenium family. Unlike Selenium RC, WebDriver does not use JavaScript injection; instead, it uses built-in support from the browser engine. This approach can make it faster, more dependable, and handle many modern web applications.
Core Functionality:
- Cross-Browser Testing: WebDriver works with multiple browser compatibility, and it supports Chrome, Firefox, Edge, Safari, and Opera.
- Programming Language Support: It is available for different languages: Java, Python, C#, Ruby, JavaScript, Kotlin, and more.
- Headless Browser Execution: This enables the running of tests without necessarily having to open a graphical user interface with the browser, which increases performance and enables CICD integration.
- Advanced Interaction Capabilities: This feature allows multiple user interactions of a certain difficulty level, such as drag-and-drop, mouse-over, and keyboard listening.
Selenium WebDriver Architecture
Understanding Selenium WebDriver’s architecture is crucial to leverage its full potential. It comprises the following components:
- Language Bindings Selenium provides language-specific bindings (libraries) for test scripts written in Java, Python, C#, etc. These bindings communicate with the browser driver.
- JSON Wire Protocol WebDriver uses the JSON Wire Protocol to send HTTP requests and interact with browsers. This protocol makes communication between test scripts and browser drivers easier.
- Browser-specific drivers like ChromeDriver and GeckoDriver are intermediaries between the WebDriver and the browser. They translate JSON commands into browser-native commands.
- Selenium WebDriver is compatible with multiple browser names, including Google Chrome, Mozilla Firefox, Safari, Microsoft Edge, and Opera. It can be classified into local tests and remote tests.
What is Selenium?
Before diving deeper into Selenium WebDriver’s features, it is important to address the question, what is Selenium? Selenium is a suite of tools designed for automating web browser interactions. It supports multiple languages and frameworks, making it an essential choice for web application testing.
Setting Up Selenium WebDriver
When it comes to testing online applications, Selenium WebDriver is perhaps the most useful tool. However, these steps must be taken in order to set it up:
Step 1: Install a Programming Language
Choose a programming language supported by Selenium, such as Python or Java. Install the required runtime environment and IDE.
Step 2: Install WebDriver Bindings
For Python, install the Selenium package using pip:
pip install Selenium
Step 3: Download Browser Driver
Download and configure the appropriate browser driver (e.g., ChromeDriver) and ensure it is accessible through the system’s PATH.
Step 4: Write Your First Script
Example (Python):
from selenium import webdriver
# Launch Browser
driver = webdriver.Chrome()
driver.get(“https://example.com”)
# Interact with Elements
element = driver.find_element(“name”, “q”)
element.send_keys(“Selenium WebDriver”)
element.submit()
# Close Browser
driver.quit()
Key Features of Selenium WebDriver
One of the most widely used tools for testing online applications is undoubtedly Selenium WebDriver. Its key features include:
- Cross-Browser Compatibility
WebDriver supports multiple browsers, enabling tests to run consistently across platforms. This feature ensures that web applications work as expected for different users.
- Multi-Language Support
With bindings for Java, Python, C#, Ruby, and JavaScript, WebDriver integrates seamlessly with existing development workflows.
- Element Locators
WebDriver provides powerful methods for locating web elements, including:
- ID: find_element_by_id()
- Name: find_element_by_name()
- Class Name: find_element_by_class_name()
- Tag Name: find_element_by_tag_name()
- CSS Selector: find_element_by_css_selector()
- XPath: find_element_by_xpath()
- Handling Dynamic Web Elements
WebDriver can interact with elements that dynamically change or load using techniques like explicit waits and implicit waits.
from Selenium.webdriver.common.by import By
from Selenium.webdriver.support.ui import WebDriverWait
from Selenium.webdriver.support import expected_conditions as EC
wait = WebDriverWait(driver, 10)
element = wait.until(EC.presence_of_element_located((By.ID, “dynamicElement”)))
- JavaScript Execution
WebDriver allows executing JavaScript code directly within the browser:
driver.execute_script(“alert(‘Hello World!’)”)
- Handling Alerts and Pop-ups
Selenium WebDriver can handle browser alerts and pop-ups effectively:
alert = driver.switch_to.alert
alert.accept() # Accept the alert
- Screenshots and Logging
Capture screenshots for debugging and reporting purposes:
driver.save_screenshot(“screenshot.png”)
- Headless Testing
WebDriver supports headless mode for faster execution without a GUI:
from Selenium.webdriver.chrome.options import Options
options = Options()
options.add_argument(“–headless”)
driver = webdriver.Chrome(options=options)
- Mobile Testing Support
Using tools like Appium, WebDriver can automate mobile browsers and hybrid applications.
- Parallel Execution
Integrate Selenium Grid for distributed test execution, reducing overall testing time.
Advanced Concepts in Selenium WebDriver
Selenium WebDriver offers several advanced concepts to handle complex testing scenarios effectively. Here are the key advanced concepts:
- Page Object Model (POM)
Definition: A design pattern that enhances test maintenance and reduces code duplication.
Key Features:
- Creates separate classes for each web page.
- Stores locators and methods specific to each page.
- Promotes code reusability and readability.
- Data-Driven Testing (Continued)
Implementation:
- Integrates with tools like Apache POI for Excel file handling or CSV readers for external data sources.
- Popular testing frameworks such as TestNG & JUnit are said to support parameterization for data-driven tests.
Example:
@DataProvider(name = “testData”)
public Object[][] getData() {
return new Object[][] { {“user1”, “pass1”}, {“user2”, “pass2”} };
}
@Test(dataProvider = “testData”)
public void loginTest(String username, String password) {
driver.findElement(By.id(“username”)).sendKeys(username);
driver.findElement(By.id(“password”)).sendKeys(password);
driver.findElement(By.id(“login”)).click();
}
- Keyword-Driven Testing
Definition: Uses keywords to represent actions, making the framework more straightforward to use for non-programmers.
Implementation:
- Keywords like Click, EnterText, and VerifyTitle are mapped to specific WebDriver commands.
- Test scripts are executed based on these predefined keywords.
- Parallel Test Execution
Definition: Runs multiple tests simultaneously to reduce execution time.
Implementation:
- Supported by TestNG and Selenium Grid for distributed testing.
Example TestNG Configuration:
<suite name=”ParallelTestSuite” parallel=”tests” thread-count=”3″>
<test name=”Test1″>
<classes>
<class name=” tests.TestClass1″/>
</classes>
</test>
<test name=”Test2″>
<classes>
<class name=” tests.TestClass2″/>
</classes>
</test>
</suite>
- Handling Alerts, Frames, and Windows
Alerts: Use the Alert interface to navigate between alert windows.
Frames: Navigate between frames using driver.switchTo().frame().
Windows: Handle multiple windows using driver.getWindowHandles() and switch between them.
- Explicit and Fluent Waits
Explicit Wait: Waits until a specific condition is met.
Fluent Wait: Polls the DOM at intervals until the condition is met.
Example:
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“elementId”)));
- Browser Profiling and Options
Definition: Customize browser settings such as extensions, proxies, and preferences.
Example (ChromeOptions):
ChromeOptions options = new ChromeOptions();
options.addArguments(“–headless”);
options.addArguments(“–disable-gpu”);
WebDriver driver = new ChromeDriver(options);
- Handling Dynamic Elements
It uses XPath, CSS Selectors, and dynamic locators to dynamically handle web elements that change IDs or attributes.
Example XPath:
driver.findElement(By.xpath(“//input[contains(@id,’dynamicId’)]”));
- Capturing Screenshots
Definition: Captures screenshots for debugging or reporting purposes.
Example:
File screenshot = ((TakesScreenshot)driver).getScreenshotAs(OutputType.FILE);
FileUtils.copyFile(screenshot, new File(“path/to/save/screenshot.png”));
- Integration with CI/CD Tools
Definition: It can be integrated with different CI/CD systems, including Jenkins, GitLab CI/CD, or Azure DevOps, for continuous testing and deployment.
These rather sophisticated ideas are useful when constructing powerful yet largely stable test automation frameworks using Selenium WebDriver.
Best Practices for Selenium WebDriver
Here are some best practices for Selenium WebDriver to ensure efficient, maintainable, and reliable test automation:
- Use Explicit Waits
Avoid using hard-coded sleep (like Thread.sleep()), as they introduce unnecessary delays. Instead, use explicit waits to wait for elements to appear or become intractable. This approach is more reliable and reduces test execution time.
Explicit Wait Example:
For instance, when an element is responsive or static, an implicit wait waits for a predetermined amount of time to pass.
WebDriverWait(driver, 10).until(EC.element_to_be_clickable((By.ID, ‘submit_button’)))
Hence, by using an explicit wait, the tests are more robust against different network load times or speed factors.
- Page Object Model (POM)
The Page Object Model is a design pattern that allows the identification and creation of well-structured test code. In POM, every application page is mapped to a separate class with methods that primarily perform actions on the elements of the corresponding page.
Advantages of POM:
- Separation of concerns: Test scripts focus only on the test logic, while page classes handle interactions with the UI.
- Reusability: Methods to interact with UI elements are reused across tests.
- Maintainability: Changes to the UI only require changes in the page object, not in every test.
Example:
class LoginPage:
def __init__(self, driver):
self.driver = driver
self.username_field = driver.find_element(By.ID, ‘username’)
self.password_field = driver.find_element(By.ID, ‘password’)
def login(self, username, password):
self.username_field.send_keys(username)
self.password_field.send_keys(password)
self.driver.find_element(By.ID, ‘login_button’).click()
- Avoid Absolute XPaths
Absolute XPaths are fickle and likely to break if the webpage’s structure changes. Choose relative XPath or CSS selectors instead, as they are more adaptable and manageable.
Example of Absolute XPath:
- /html/body/div[1]/div[2]/input
Example of Relative XPath:
- //input[@id=’username’]
Why use Relative XPath or CSS selectors?
- They are more readable and less brittle.
- Easier to maintain, as changes in the page layout don’t often affect them.
- Handle Exceptions Gracefully
To keep the tests from failing abruptly and to offer useful error messages, it is essential to handle exceptions such as NoSuchElementException, TimeoutException, or StaleElementReferenceException.
Try-Catch Example:
try:
driver.find_element(By.ID, ‘login_button’).click()
except NoSuchElementException as e:
print(“Element not found:”, e)
It makes tests more robust and allows you to take corrective actions or capture logs when errors occur.
- CI/CD Integration
Suppose you are integrating the CI/CD pipeline to automate your tests. In that case, you can detect a defect in the early stages of your development process since tests run automatically with each change made to the code.
Tools for CI/CD:
- Jenkins
- GitLab CI/CD
- CircleCI
As previously mentioned, these tools allow Selenium tests to be automatically executed whenever code is pushed to a version control system like Git. They also give feedback in real time and make software development much faster.
- Version Compatibility
Ensure your Selenium WebDriver, browser drivers (e.g., ChromeDriver, GeckoDriver), and browsers are up-to-date. Mismatched versions can cause compatibility issues and unexpected test failures.
For Example:
If you’re using Chrome 100, you should ensure you have the corresponding ChromeDriver version that supports it.
This practice prevents errors such as SessionNotCreatedException and ensures your tests run smoothly on the latest browser versions.
- Parallel Execution
Running tests in parallel across multiple environments can drastically reduce execution time, especially for large test suites.
Selenium Grid:
- Selenium Grid allows you to distribute tests across multiple machines, enabling parallel execution. It helps to save time and resources.
Tools for Parallel Execution:
- TestNG or JUnit (with parallel execution configuration)
- Selenium Grid
- Docker for isolated test environments
For teams looking to scale their Selenium testing, LambdaTest is an AI-powered test orchestration and execution platform that offers a cloud grid with parallel test execution capabilities. It allows you to distribute tests across multiple machines and run them concurrently, saving valuable time and resources while maintaining test reliability across browsers and operating systems.
- Headless Browsing
Headless browsers are faster and consume fewer resources because they don’t require a GUI to run the tests.
Headless Mode Example with Chrome:
options = webdriver.ChromeOptions()
options.add_argument(‘–headless’)
driver = webdriver.Chrome(options=options)
It is handy, especially in CI/CD processes and cloud service provision, where an interface is not required when running tests.
Tech Blaster
Conclusion
Selenium WebDriver is one of the most popular and flexible tools for performing web drawing and testing across different browsers, languages, and testing frameworks. Due to aspects such as the above, it possesses a rich feature set and is extensible, and therefore, it is widely used by developers and testers. When people clearly grasp what the test automation framework is made of, what it contains, and how it should be done, it is possible to design a solid, large-scale, and sustainable test automation solution.
In a nutshell, Selenium WebDriver contains everything a starting and a professional tester might address to face modern challenges. Learn how to automate tests using Selenium WebDriver so that your customers have an enjoyable experience with your web applications!